Getting Started with Livewire Components

Livewire is a powerful framework that simplifies the process of building dynamic and interactive user interfaces for your web applications. By leveraging Livewire, you can create reusable components that handle complex interactions with ease. In this comprehensive guide, we will take you through the process of creating Livewire components, from understanding the basics to advanced techniques.
What are Livewire Components?
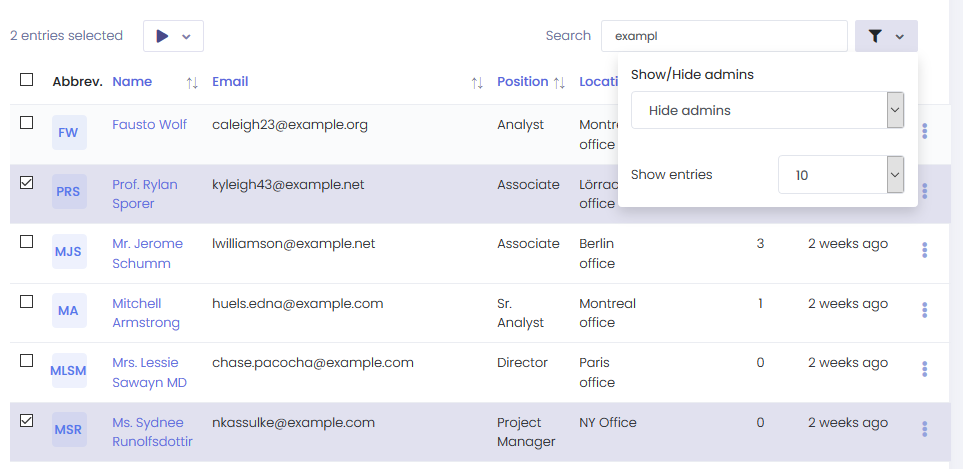
Livewire components are reusable, self-contained pieces of code that encapsulate a specific functionality or user interface element. They are designed to make your application more modular and maintainable by breaking down complex interfaces into smaller, manageable parts. Livewire components are rendered on the server-side and updated in real-time, providing a seamless user experience.
Setting Up Your Environment
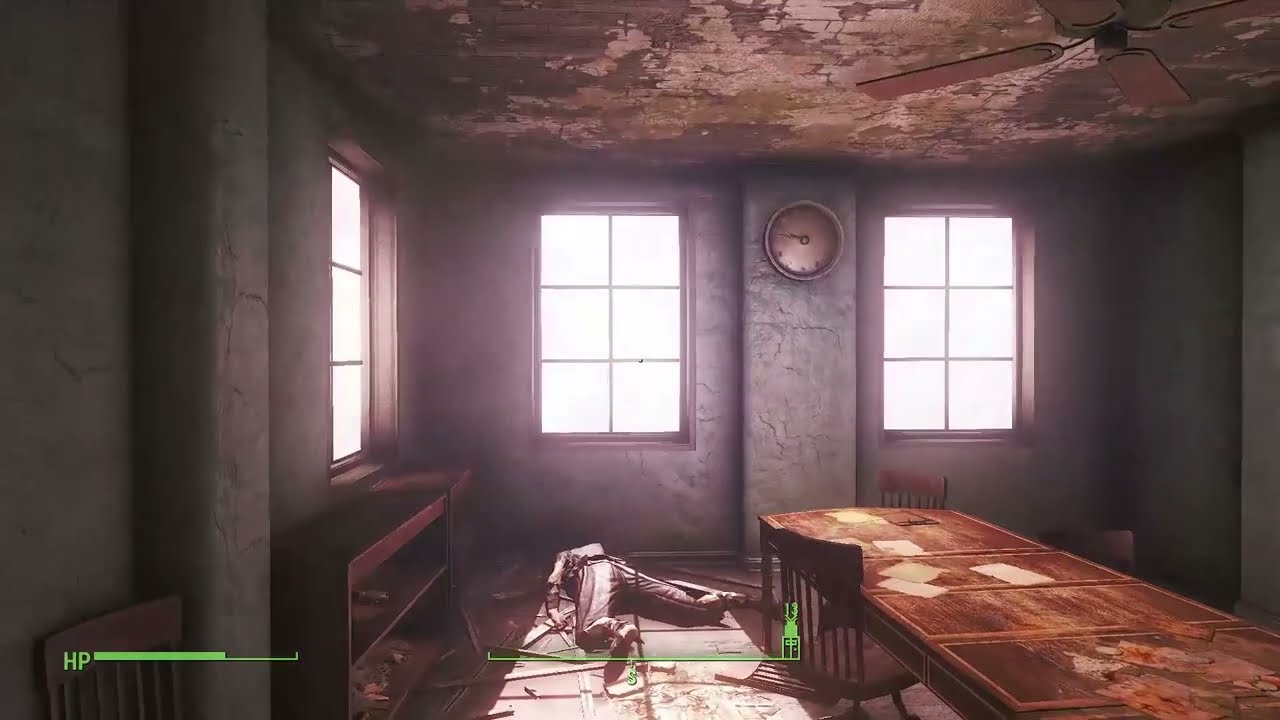
Before we dive into creating Livewire components, ensure you have the following prerequisites in place:
- A modern web browser (Chrome, Firefox, Safari, etc.)
- A code editor of your choice (VS Code, Sublime Text, etc.)
- PHP installed on your local machine
- A web server (Apache, Nginx) with PHP support
- Composer installed (for dependency management)
Once you have the prerequisites, follow these steps to set up your development environment:
- Create a new project directory and navigate to it using your terminal.
- Initialize a new Composer project by running
composer init
. - Install Livewire using Composer:
composer require livewire/livewire
- Create a new PHP file (e.g.,
app.php
) and include the Livewire autoloader:require 'vendor/autoload.php';
- Add the following code to your
app.php
file to define a basic Livewire component:
use Livewire\Component;
class HelloWorld extends Component
{
public $name = 'Livewire';
public function render()
{
return view('hello-world', ['name' => $this->name]);
}
}
In the above code, we define a simple Livewire component named HelloWorld
that displays a greeting with a default name of 'Livewire'
. The render
method returns a view named 'hello-world'
and passes the $name
variable to it.
Creating Your First Livewire Component

Now that we have our basic setup in place, let's create our first Livewire component. We'll create a simple counter component that allows users to increment and decrement a counter value.
- Create a new PHP file named
counter.php
in your project directory. - Add the following code to define the
Counter
component:
use Livewire\Component;
class Counter extends Component
{
public $count = 0;
public function increment()
{
$this->count++;
}
public function decrement()
{
$this->count--;
}
public function render()
{
return view('counter', ['count' => $this->count]);
}
}
In the above code, we define a Counter
component with an initial count of 0
. We have two methods, increment
and decrement
, which update the $count
variable accordingly. The render
method returns a view named 'counter'
and passes the $count
variable to it.
Rendering the Component in a View

To render our Counter
component in a view, we need to create a corresponding Blade template. Blade is Laravel's templating engine, but you can use any templating engine or even plain HTML.
- Create a new Blade template file named
counter.blade.php
in your project directory. - Add the following code to the template:
Count: {{ $count }}
In the above template, we have two buttons with wire:click
directives that call the increment
and decrement
methods of our Counter
component. The {{ $count }}
blade directive displays the current count value.
Using Livewire in Your Application
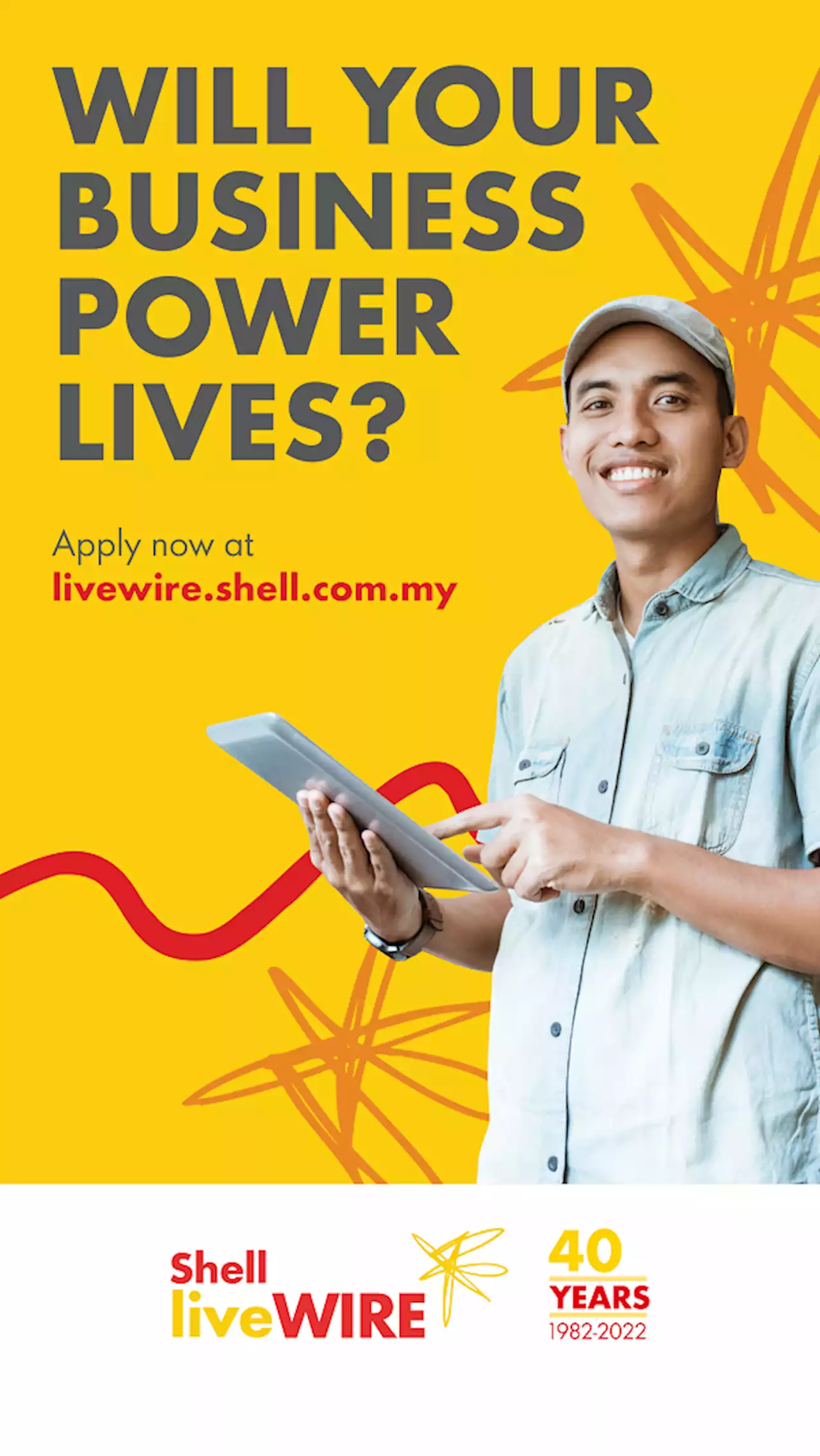
To use our Livewire components in our application, we need to integrate them into our routes or controllers. Let's assume we have a simple Laravel application with a routes/web.php
file.
- Open your
routes/web.php
file and add the following route:
use App\Http\Livewire\Counter;
Route::get('/counter', Counter::class);
In the above code, we import the Counter
component and define a route that renders the component when accessed.
Advanced Livewire Techniques
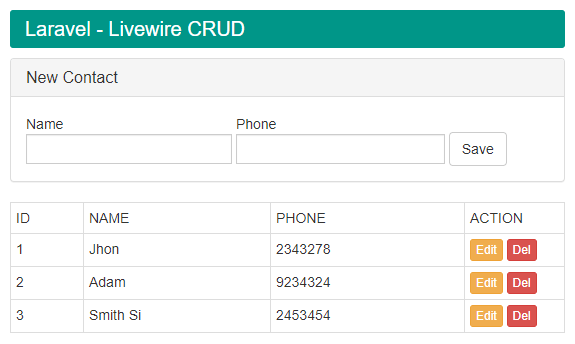
Data Validation
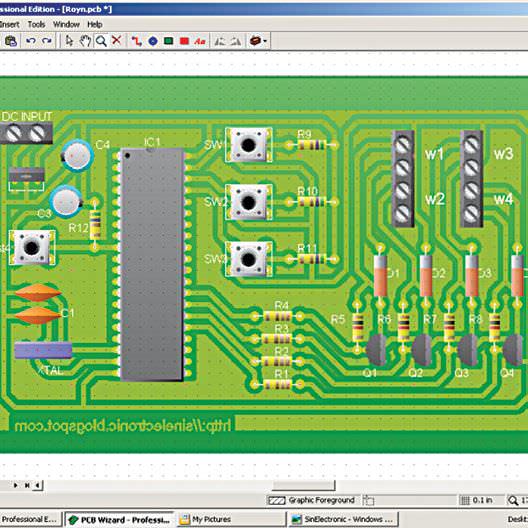
Livewire provides built-in support for data validation, allowing you to validate user input directly within your components. You can define validation rules and display error messages to the user.
use Livewire\Component;
use Illuminate\Support\Facades\Validator;
class FormComponent extends Component
{
public $name;
protected $rules = [
'name' => 'required|min:3',
];
public function updated($propertyName)
{
$this->validateOnly($propertyName);
}
public function submit()
{
$this->validate();
// Process form submission here
}
public function render()
{
return view('form');
}
}
Event Emission and Listening

Livewire allows you to emit and listen to events, enabling communication between components and triggering actions based on specific events. You can use events to update multiple components simultaneously or trigger custom actions.
use Livewire\Component;
class EventComponent extends Component
{
public $message = 'Hello, Livewire!';
public function emitEvent()
{
$this->emit('customEvent', 'Message from EventComponent');
}
public function render()
{
return view('event');
}
}
Pagination and Lazy Loading

Livewire provides powerful pagination features, allowing you to load data in smaller chunks and improve performance. You can implement lazy loading to fetch data dynamically as the user scrolls through the page.
Testing Livewire Components

Testing your Livewire components is crucial to ensure their functionality and behavior. Livewire provides a testing API that allows you to write comprehensive tests for your components.
use Livewire\Testing\TestableLivewire;
use Livewire\Testing\TestableLivewireInstance;
it('can render the component', function () {
$component = TestableLivewire::factory(Counter::class)->make();
$component->assertSee('Count: 0');
});
Conclusion

Livewire is a versatile and powerful framework that simplifies the development of dynamic and interactive web applications. By following this guide, you should now have a solid understanding of how to create Livewire components and integrate them into your projects. Remember to explore the official Livewire documentation for more advanced features and best practices.
What is Livewire, and why should I use it?

+
Livewire is a full-stack framework for Laravel that allows you to create dynamic and interactive user interfaces without writing JavaScript. It simplifies the development process by handling complex interactions on the server-side, resulting in cleaner and more maintainable code.
How do I install Livewire in my Laravel project?
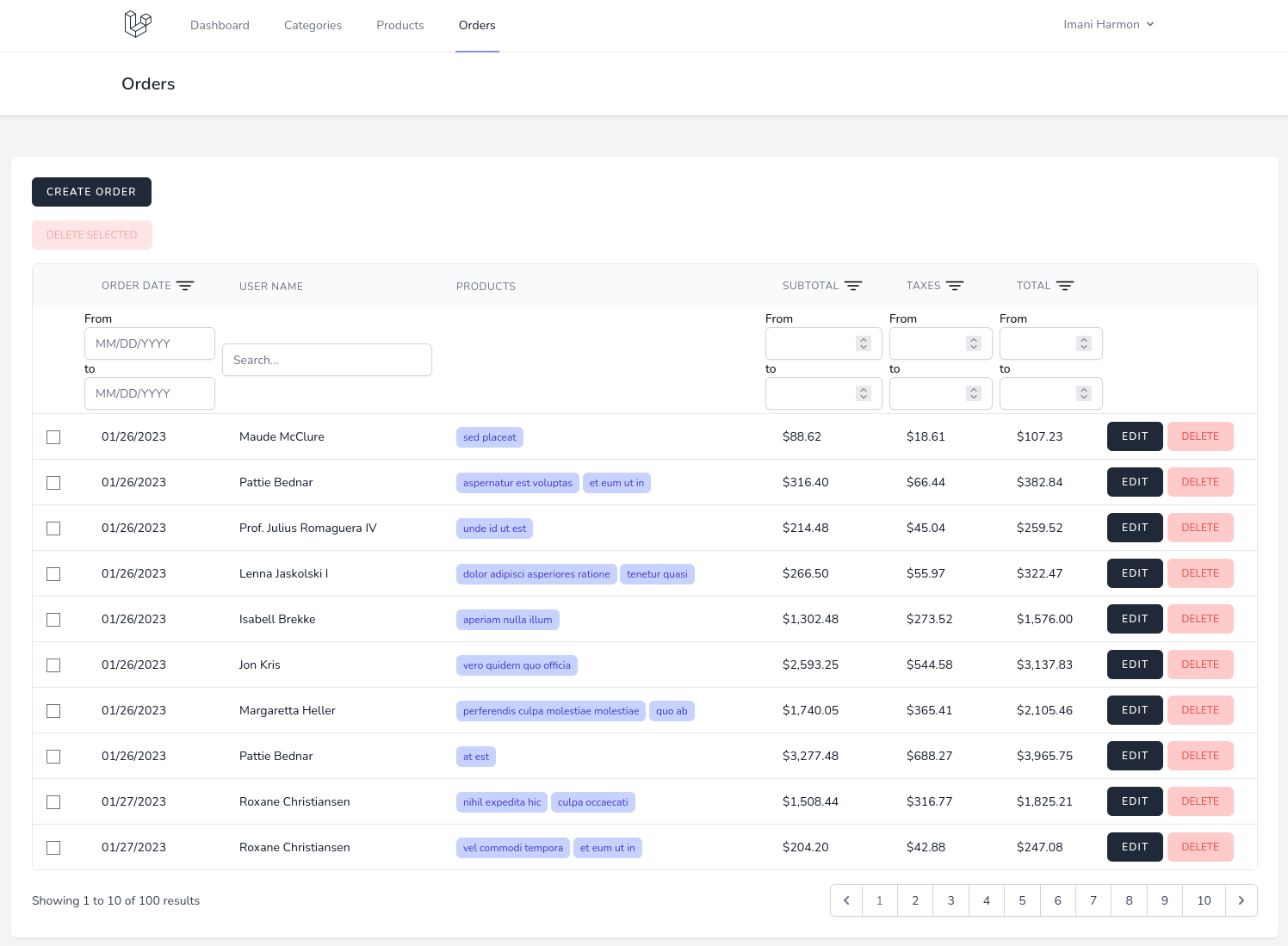
+
To install Livewire in your Laravel project, run the following command in your terminal: composer require livewire/livewire
. This will add Livewire as a dependency and make it available for use in your application.
Can I use Livewire with other PHP frameworks or plain PHP applications?

+
Yes, Livewire is not limited to Laravel. You can use it with any PHP framework or even in plain PHP applications. It provides a flexible and powerful way to create interactive components, making it a great choice for any PHP project.
What are some best practices for creating Livewire components?
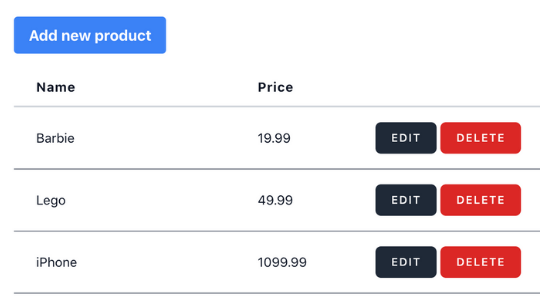
+
When creating Livewire components, it’s important to follow best practices to ensure maintainability and scalability. Some key practices include keeping components small and focused, using meaningful and descriptive names, and handling data validation and sanitization properly. Additionally, make use of Livewire’s built-in features like lazy loading and event emission to enhance performance and user experience.